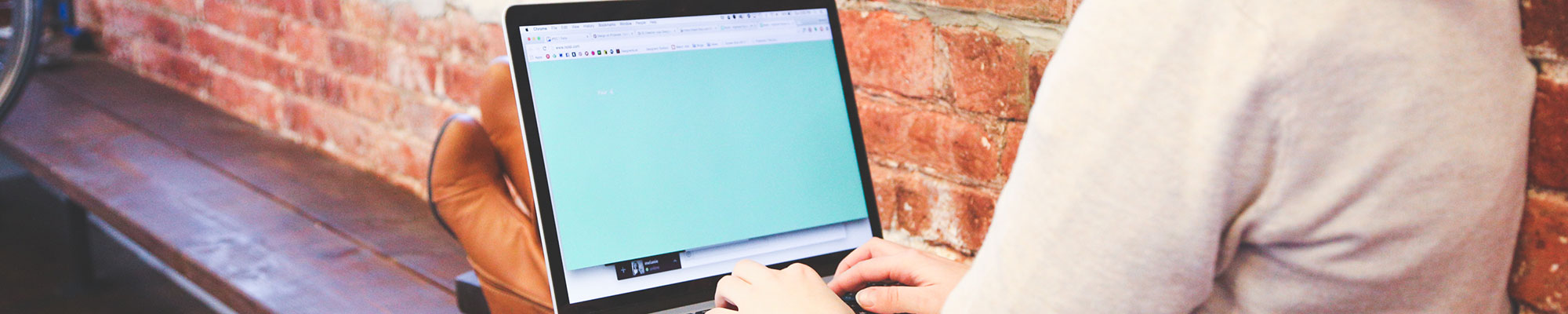
Using JavaScript with a Database
What is it?
Firebase is a web-based application that allows you to create an online database that can be accessed by your app. It is owned by Google. In this lesson, you will gain experience using JavaScript to provide get data from a database you create in Firebase.
Why should I learn it?
There are valuable sources of information all over the Web. You should learn how to access items in a realtime manner.
What can I do with it?
Your web pages can access information from a database you create. It's like your own CMS!
How long will it take?
Programming is something that you learn over time, through practice. But in a few hours, you will be introduced to the basic elements of APIs and databases that you will be able to apply to your own projects. You will need to continue to practice with these concepts to best use them.
Creating a Database
You can create your own database using Google's Firebase and access it via JavaScript. First, you must go to firebase.google.com and login with a Google account. Go to your Console, and there you can set up a New Project. Go through the steps to give it a name and create the project (you don't have to include Google Analytics). Click continue when project is ready, and then choose the icon for Web project. Register your app with a nickname (ok to be same as project) and register app. You don't need to include hosting, if you already have a hosting account.
Copy the script that allows the app to work for you and save it somewhere. We will be using it in our code. It contains your authentication information for your project. Choose Continue to Console. Any time you need to return to your projects, you can find them at the Console. We will be using the Realtime Database option. Firebase products are free for entry level services, then you can pay more as your projects scale.
Choose test mode and a location (the default is fine). Once the database is ready, you can begin adding fields and data. See examples below. I have created a few nodes in one database that we will access in different ways in the examples.
Changing Message
This example demontrates how to post a simple message to a database that is automatically displayed on a web page. The database is connected to the page and a "snapshot" of the data is made available to it. The "on" method allows it to be updated automatically from the database. Use "once" if you want the user to have to refresh to see updated info. This example gets the data from database child node named "text" and updates the DOM element "answer".
Read a List
This example uses a more complex data structure to get multiple values from a database. It uses a forEach function to grab every child value from the "venues" node. Notice that before the forEach statement, the code re-initializes the "answer" element, to not duplicate the items. This example places the items in an HTML unordered list.
More Complex Dataset
Let's say you have even more complex data, in this case, a listing of venues along with their zip codes. You could imagine having all kinds of data associated with an individual venue, like address, phone, genre, other descriptions, etc. Like the previous dataset, each item has a unique main key, but it has both name and zip for each venue. This example gets a "snapshot" of the data of each child of the location node and then gets the "name" and "zip" value of each location. This example formats the data into a table with name and zipcode.
More Complex Dataset - Filtering
This example uses the same code as above, but allows you to filter with a dropdown. Notice the getZip() function that is created to be run each time the dropdown changes.
Writing to a Database
You may want to create a system that allows users to upload items to your database. You might have administrative uses who are authorized to make updates. Or you might be creating a social network site that allows users to add content. Using the code that lists venues, we add a form that allows a user to add a venue with name and zip to the database. In the script, the push function creates a unique id for the item and writes name and zip from the form to the database. Of course, you would only want this to be for authorized users with administrative access, but this is a basic example that demonstrates how to write to a database.
Add a form to the html below the table
Add submitVenue() function below ref node creation
Moving On
Now you have a basic understanding of API and database concepts. Practice using these techniques in your projects.